Identity and Location
Identity verification and routing intelligence for telecoms and IT services.
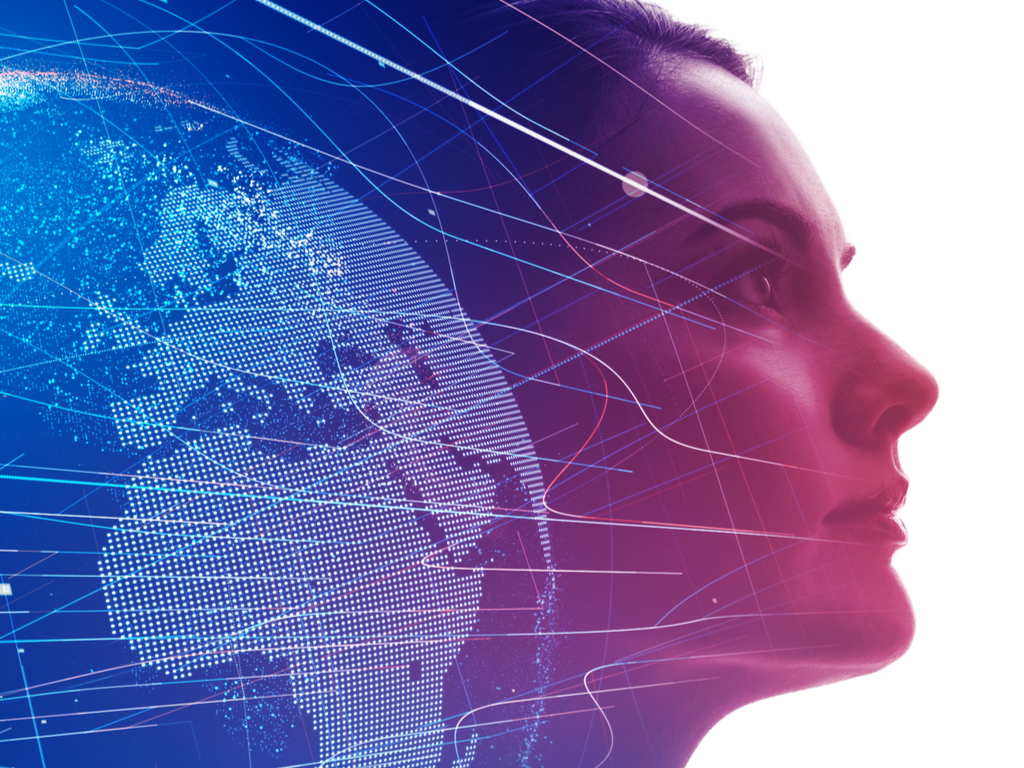
Identity verification is an essential part of online transactions between businesses and individuals. Digital identities, financial accounts, IP address and the physical address of a person often need to be associated with each other to conduct transactions of value.
Using the Service
The Melrose Labs Identity and Location service is available using the REST API.
REST API
The Melrose Labs Identity and Location service is available using our REST Location API.
Location API : Mobile Number Lookup / HLR Lookup
The Location API of the Identity and Location service can be used to lookup the mobile network of a mobile phone number. This information can provide a means of more intelligent routing of calls and messages to the mobile number.
Perform mobile number lookup using the Identity and Location service with RESTful Location API
Example using cURL, Node.js, Python, PHP
Request:
curl https://api.melroselabs.com/location/telno/447700000000 \
--header 'x-api-key: [API_KEY]'
Response:
{
"subject": "447700000000",
"number": {
"country": "44",
"ndc": "7700",
"subscriber": "000000"
},
"geo": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"network": {
"name": "Vodafone",
"mccmnc": "234-15",
"geoHome": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"geoCurrent": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
}
}
}
Request:
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.melroselabs.com/location/telno/447700000000',
'headers': {
'x-api-key': '[API_KEY]'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body); // response is of type application/json
});
Response:
{
"subject": "447700000000",
"number": {
"country": "44",
"ndc": "7700",
"subscriber": "000000"
},
"geo": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"network": {
"name": "Vodafone",
"mccmnc": "234-15",
"geoHome": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"geoCurrent": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
}
}
}
Request:
import requests
import json
url = "https://api.melroselabs.com/location/telno/447700000000"
headers = {
'x-api-key': '[API_KEY]'
}
response = requests.request("POST", url, headers=headers)
# response is of type application/json
print(response.text.encode('utf8'))
Response:
{
"subject": "447700000000",
"number": {
"country": "44",
"ndc": "7700",
"subscriber": "000000"
},
"geo": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"network": {
"name": "Vodafone",
"mccmnc": "234-15",
"geoHome": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"geoCurrent": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
}
}
}
Request:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.melroselabs.com/location/telno/447700000000",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_HTTPHEADER => array(
"x-api-key: [API_KEY]" )
));
$response = curl_exec($curl);
curl_close($curl);
echo $response; // response is of type application/json
?>
Response:
{
"subject": "447700000000",
"number": {
"country": "44",
"ndc": "7700",
"subscriber": "000000"
},
"geo": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"network": {
"name": "Vodafone",
"mccmnc": "234-15",
"geoHome": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
},
"geoCurrent": {
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU"
}
}
}
Location API : IP Lookup
The Location API of the Identity and Location service can be used to lookup the geographic location of an IP address.
Perform IP lookup using the Identity and Location service with RESTful Location API
Example using cURL, Node.js, Python, PHP
Request:
curl https://api.melroselabs.com/location/ip/194.247.82.140 \
--header 'x-api-key: [API_KEY]'
Response:
{
"subject": "194.247.82.140",
"geo": {
"city": "Edinburgh",
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU",
"latitude": "55.543",
"longitude": "-3.141",
"timezone": "GMT",
"weather_code": "UKXX0052",
"postal_code": "EH3 8LB"
}
}
Request:
var request = require('request');
var options = {
'method': 'POST',
'url': 'https://api.melroselabs.com/location/ip/194.247.82.140',
'headers': {
'x-api-key': '[API_KEY]'
}
};
request(options, function (error, response) {
if (error) throw new Error(error);
console.log(response.body); // response is of type application/json
});
Response:
{
"subject": "194.247.82.140",
"geo": {
"city": "Edinburgh",
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU",
"latitude": "55.543",
"longitude": "-3.141",
"timezone": "GMT",
"weather_code": "UKXX0052",
"postal_code": "EH3 8LB"
}
}
Request:
import requests
import json
url = "https://api.melroselabs.com/location/ip/194.247.82.140"
headers = {
'x-api-key': '[API_KEY]'
}
response = requests.request("POST", url, headers=headers)
# response is of type application/json
print(response.text.encode('utf8'))
Response:
{
"subject": "194.247.82.140",
"geo": {
"city": "Edinburgh",
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU",
"latitude": "55.543",
"longitude": "-3.141",
"timezone": "GMT",
"weather_code": "UKXX0052",
"postal_code": "EH3 8LB"
}
}
Request:
<?php
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.melroselabs.com/location/ip/194.247.82.140",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_HTTPHEADER => array(
"x-api-key: [API_KEY]" )
));
$response = curl_exec($curl);
curl_close($curl);
echo $response; // response is of type application/json
?>
Response:
{
"subject": "194.247.82.140",
"geo": {
"city": "Edinburgh",
"country_name": "United Kingdom",
"country_code": "GB",
"continent": "Europe",
"continent_code": "EU",
"latitude": "55.543",
"longitude": "-3.141",
"timezone": "GMT",
"weather_code": "UKXX0052",
"postal_code": "EH3 8LB"
}
}
Get your API Key now and start using the Identity and Location service REST API
SIGN-UP | LOGIN TO GET API KEYPricing
Contact us for Melrose Labs Identity and Location pricing.
Data Retention and Data Privacy
Data retention and data privacy policies ensure that data is handled securely and in accordance with GDPR. Mobile telephone numbers and message content are kept encrypted at rest and in motion whenever possible.
Service snapshot
- Verify identity using mobile number and email address
- Lookup geographic location using IP address
- Lookup mobile network using telephone number
- HLR lookup